SwiftUI Localization
I need to implement manual language switching in the app settings, which requires additional coding for Swift Localizable.
- Interface: SwiftUI
- Language: Swift5.9
- Xcode: 15
As you can see in the image, the text changes when the user selects a different language.
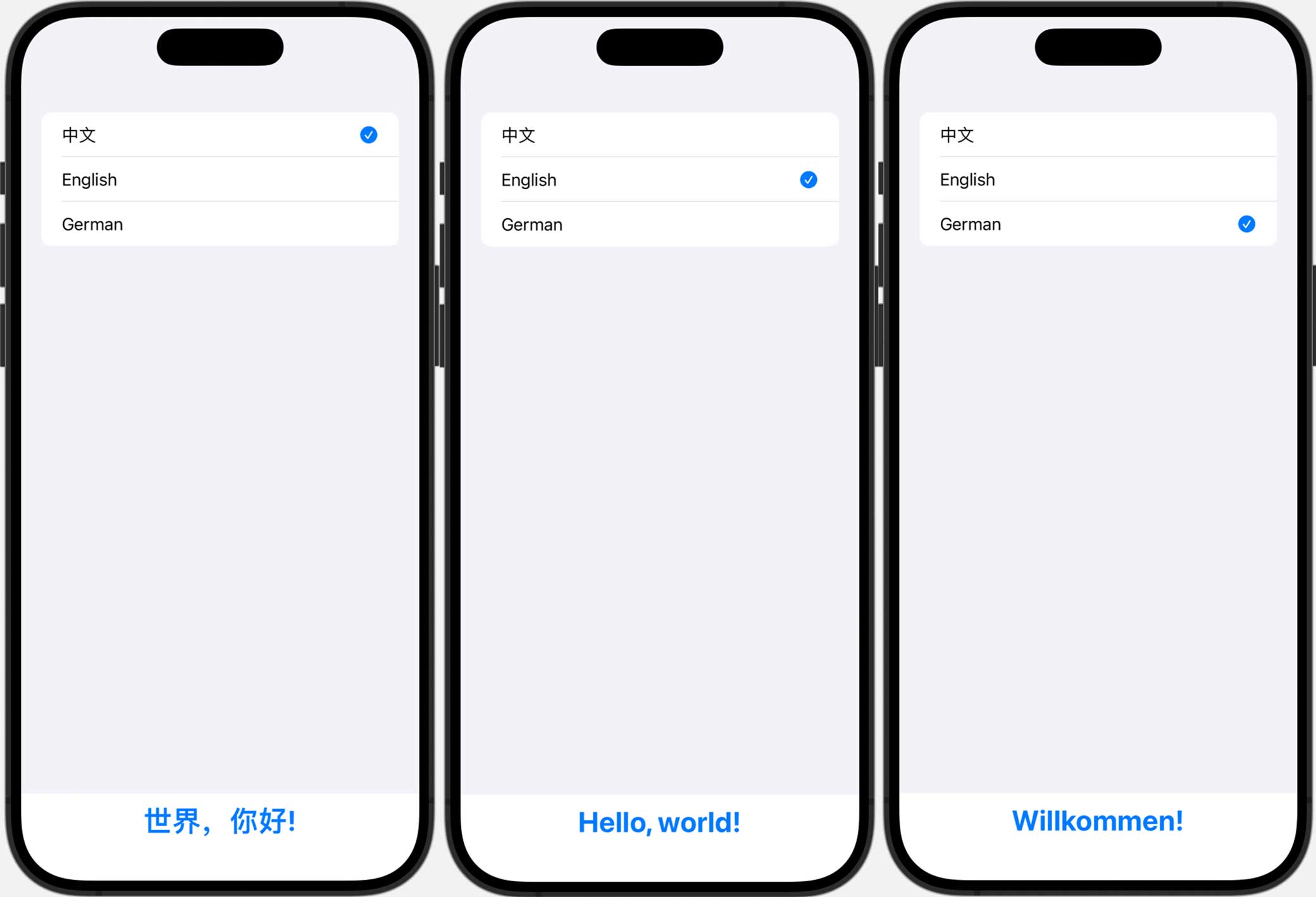
Operation
1. Create Localizable files
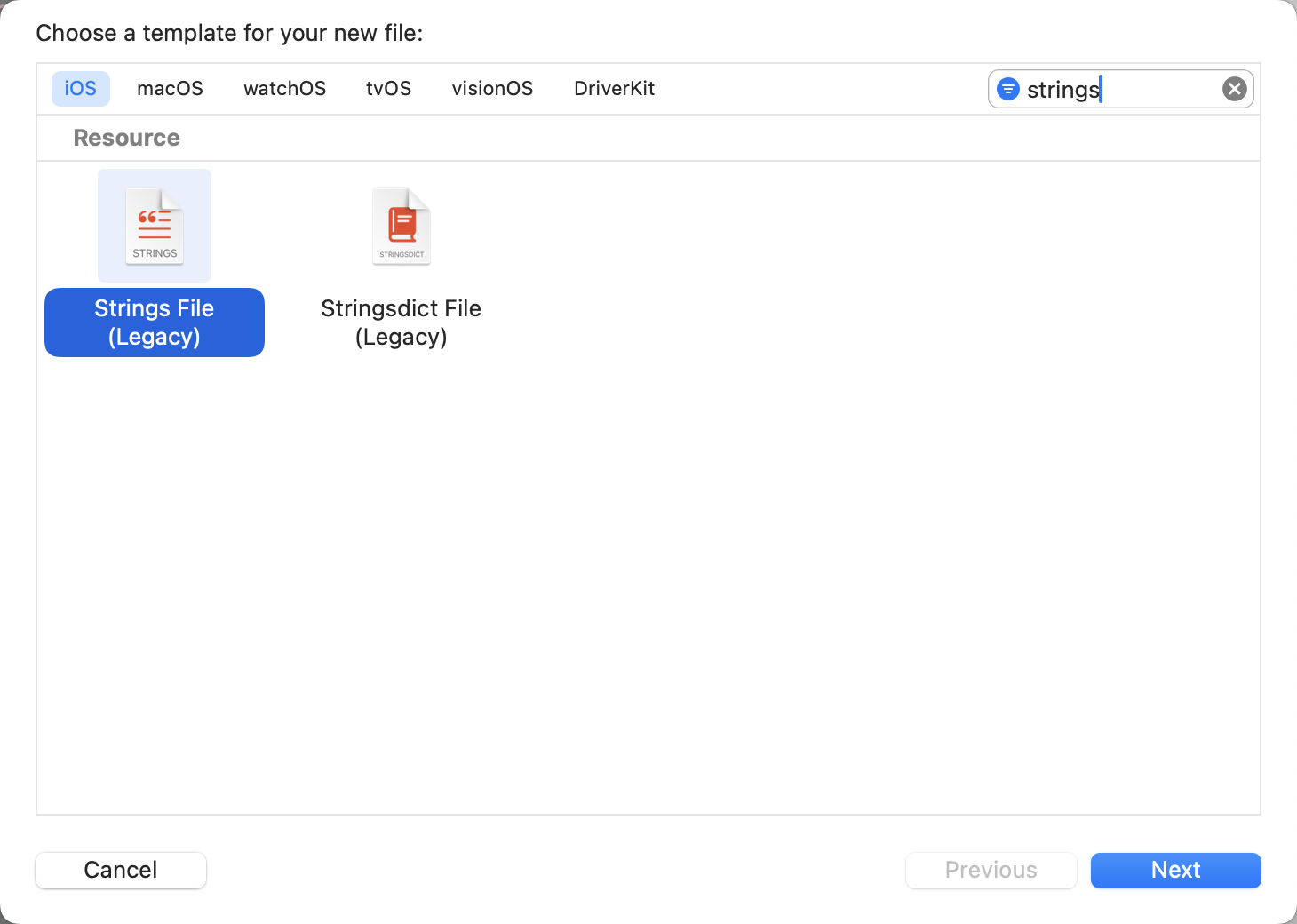
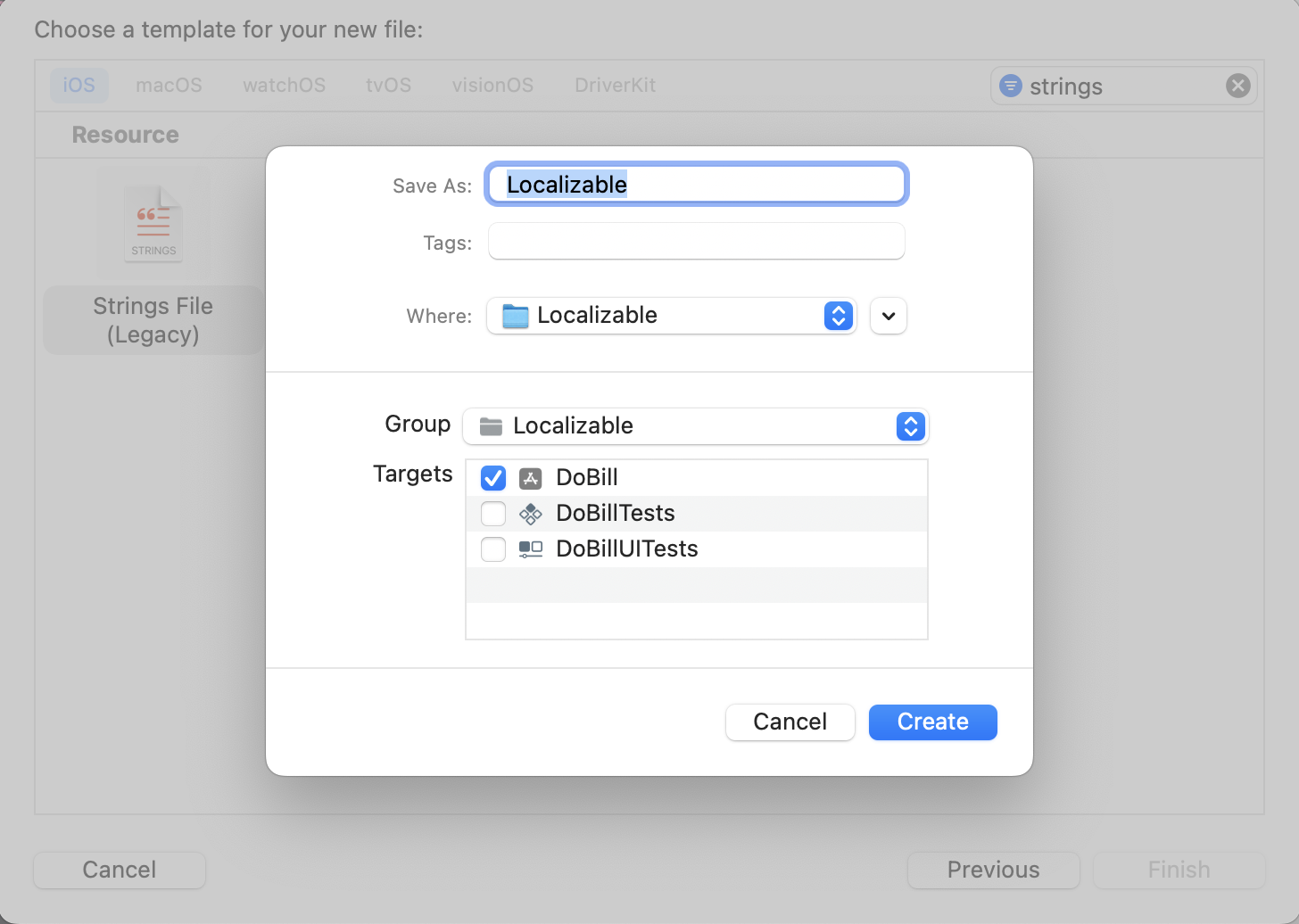
2. Add Languages
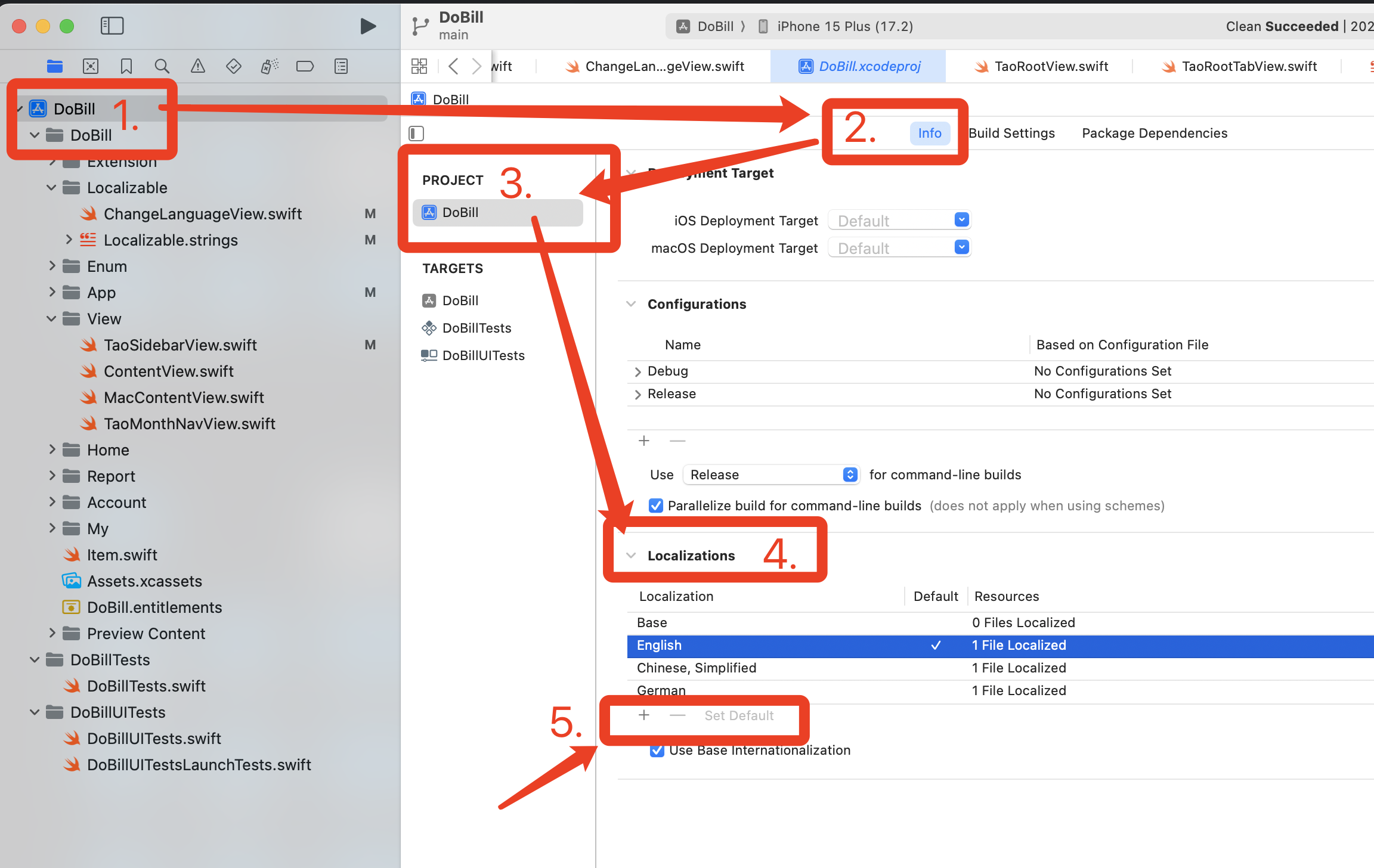
3. Localization
On the right side, click Localize...
A pop-up window will appear. And then click Localize
to continue.
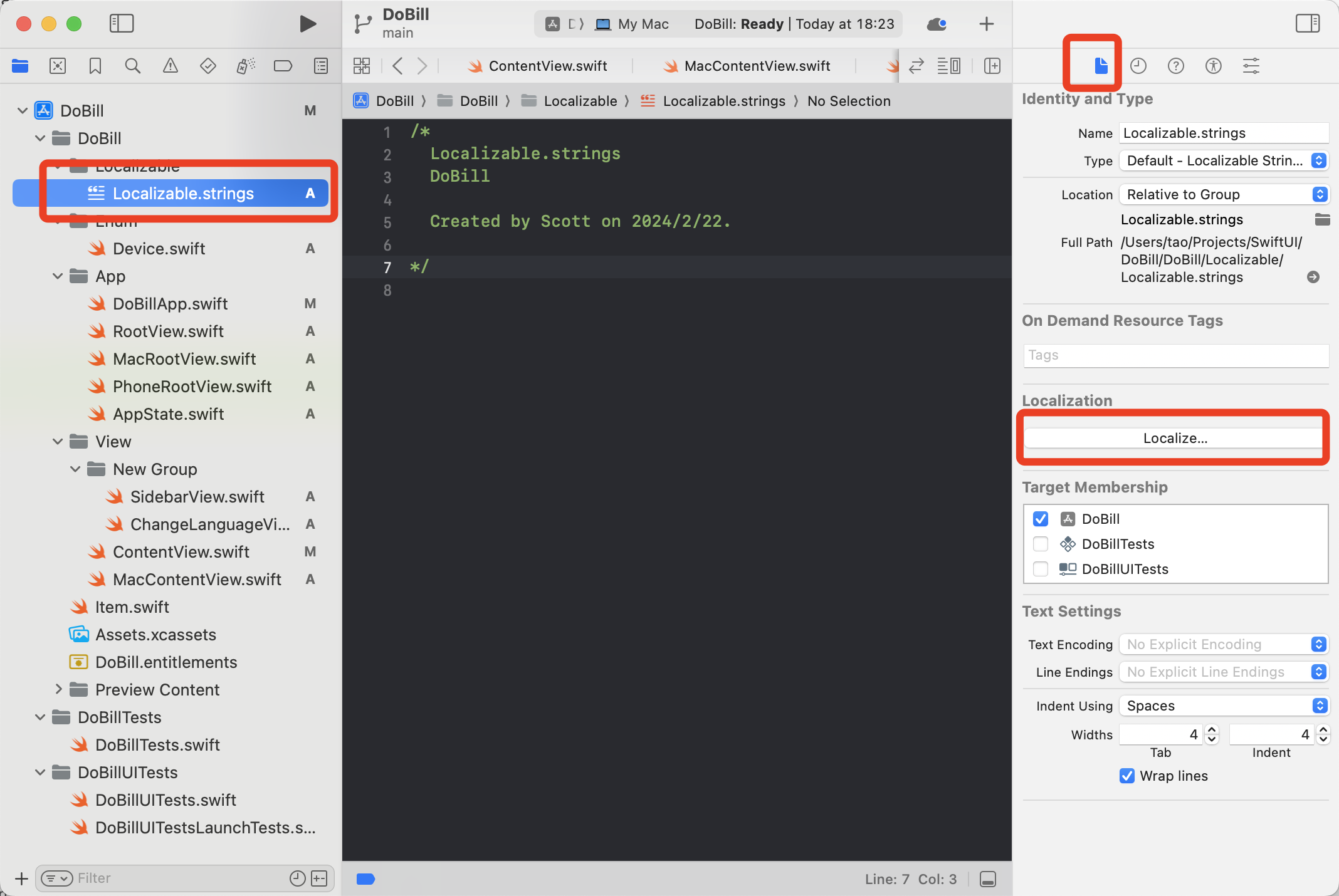
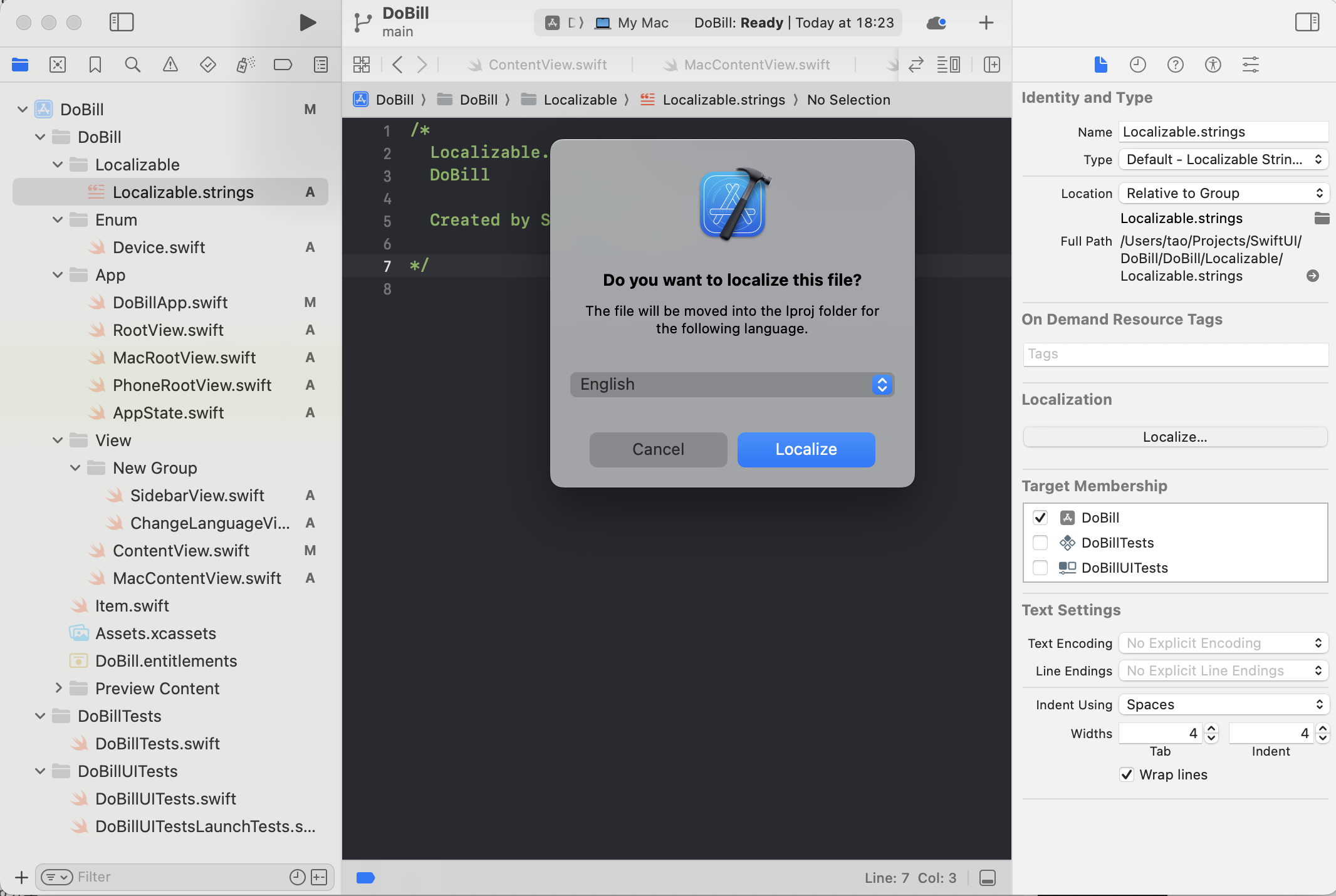
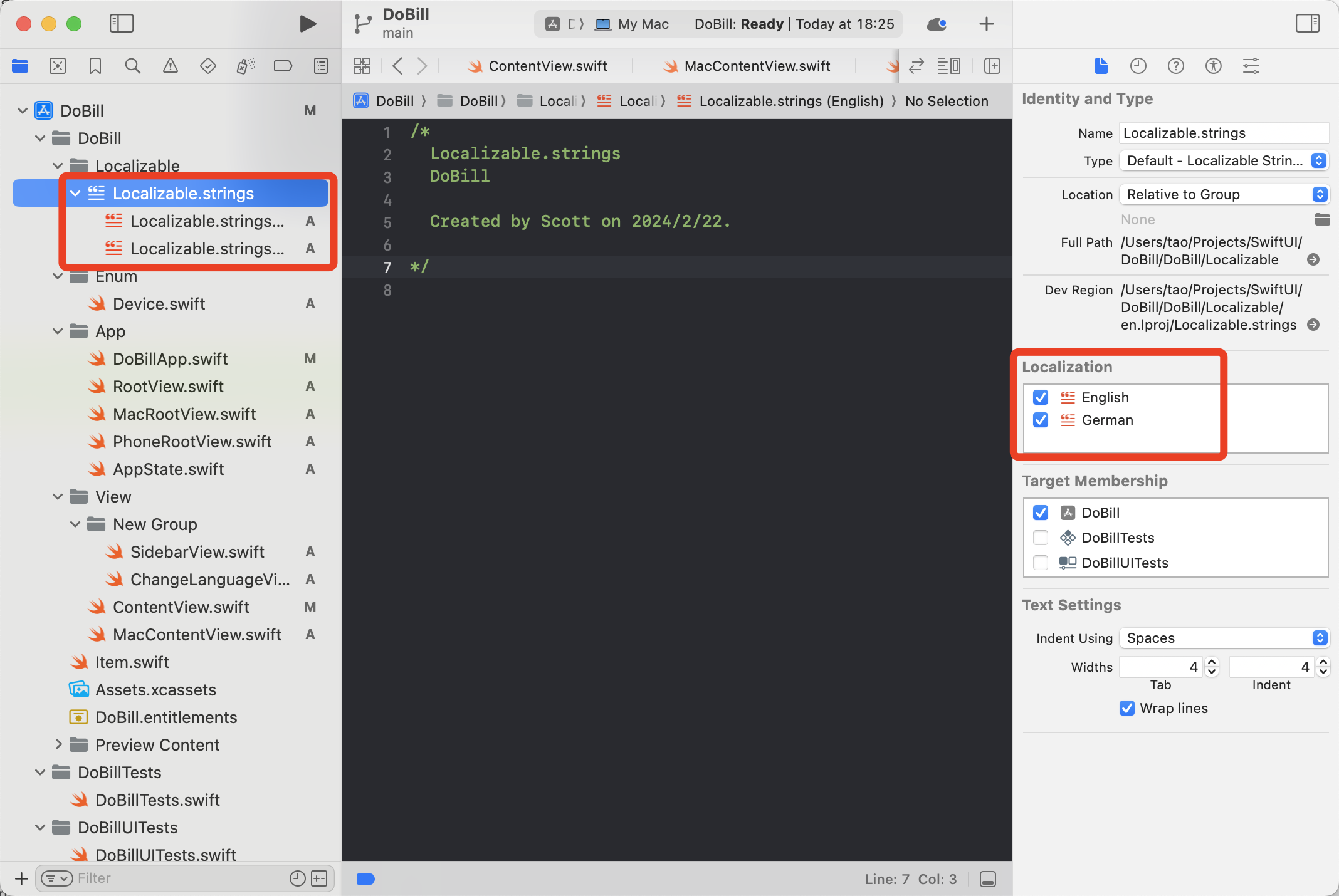
AppState.swift
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
import Foundation
import SwiftUI
final class TaoAppState: ObservableObject {
@AppStorage("language") var language = "en"
}
extension String {
var localized: String {
let res = UserDefaults.standard.string(forKey: "language")
let path = Bundle.main.path(forResource: res, ofType: "lproj")
let bundle: Bundle
if let path = path {
bundle = Bundle(path: path) ?? .main
} else {
bundle = .main
}
return NSLocalizedString(self, bundle: bundle, value: "", comment: "")
}
}
|
ChanageLanguageView.swift
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
|
import SwiftUI
struct ChangeLanguageView: View {
@EnvironmentObject private var appState: AppState
private let languages = [
"English": "en",
"German": "de",
"中文": "zh-Hans"
]
var body: some View {
VStack {
List {
ForEach(Array(languages.keys), id: \.self) { v in
LabeledContent(v) {
Image(systemName: "checkmark.circle.fill")
.foregroundColor(.accentColor)
.opacity(isSelected(v) ? 1 : 0)
}
.contentShape(Rectangle())
.onTapGesture {
if isSelected(v) { return }
appState.language = languages[v]!
}
}
}
Text("hello".localized)
.font(.title)
.fontWeight(.bold)
.foregroundStyle(.blue)
Spacer()
}
}
private func isSelected(_ language: String) -> Bool {
appState.language == languages[language]
}
}
#Preview {
ChangeLanguageView()
.environmentObject(AppState())
}
|