From Apple Document If your app has a minimum deployment target of iOS 16, iPadOS 16, macOS 13, tvOS 16, or watchOS 9, or later, transition away from using NavigationView. In its place, use NavigationStack and NavigationSplitView instances.
I need a list of Collections, and the custom Cell, then with NavigationStack
link to the next View.
Demo Download.zip
CardView
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
|
import SwiftUI
struct CardView: View {
let title: String
let color: Color = .random
var body: some View {
VStack {
RoundedRectangle(cornerRadius: 12).foregroundColor(color)
Text(title)
.font(.title2)
}
}
}
struct CardView_Previews: PreviewProvider {
static var previews: some View {
CardView(title: "Hello world")
}
}
extension Color {
static var random: Color {
return Color(
red: .random(in: 0...1),
green: .random(in: 0...1),
blue: .random(in: 0...1)
)
}
}
struct MockStore {
static var cards = [
Card(title: "Sun"),
Card(title: "Mercury"),
Card(title: "Venus"),
Card(title: "Earth"),
Card(title: "Mars"),
Card(title: "Jupiter"),
Card(title: "Saturn"),
Card(title: "Uranus"),
Card(title: "Neptune"),
Card(title: "Pluto"),
Card(title: "Solar System"),
Card(title: "Galaxy"),
Card(title: "Universe"),
Card(title: "Remote Antiquity"),
Card(title: "Foreworld"),
]
}
struct Card: Identifiable, Hashable {
let id = UUID()
let title: String
func hash(into hasher: inout Hasher) {
hasher.combine(id)
// hasher.combine(title)
}
}
|
View |
NavigationStack |
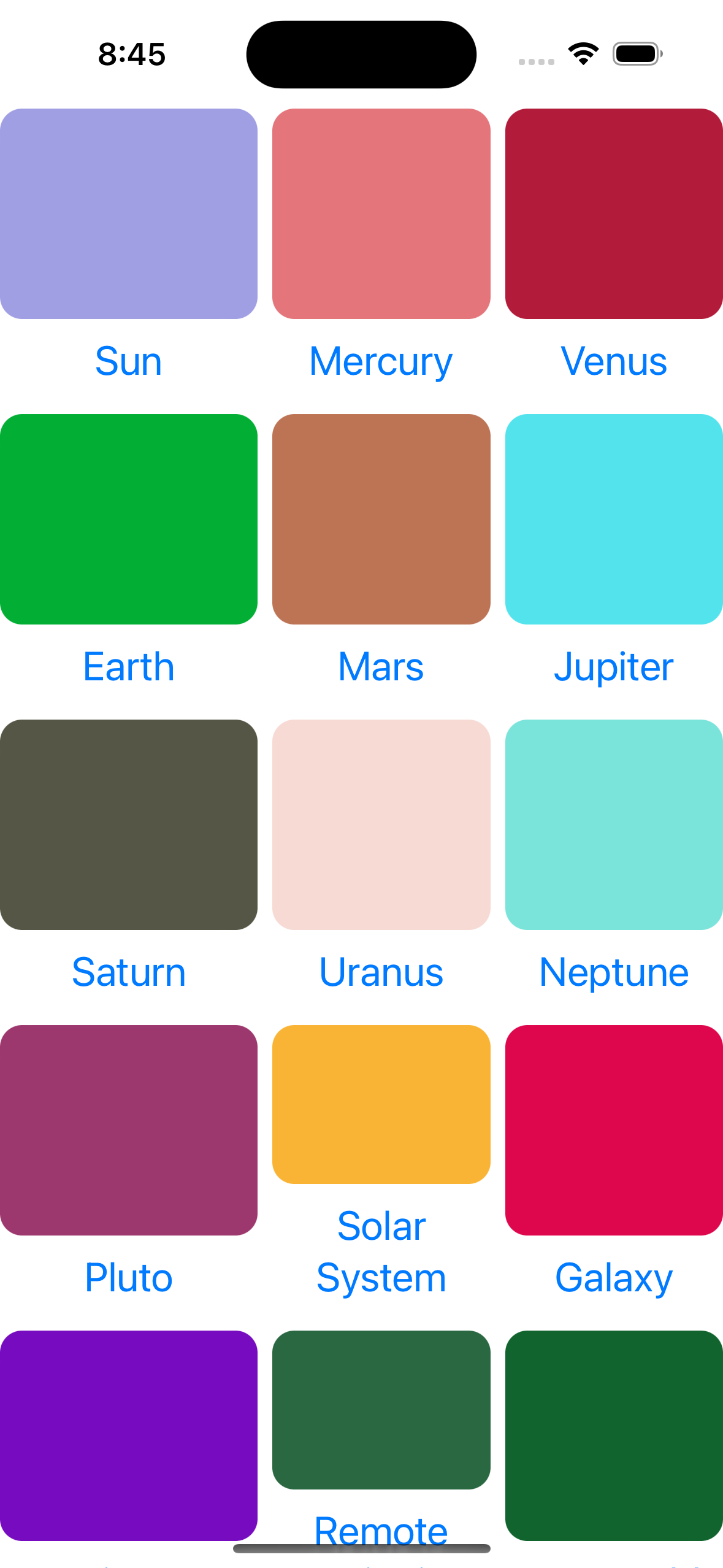 |
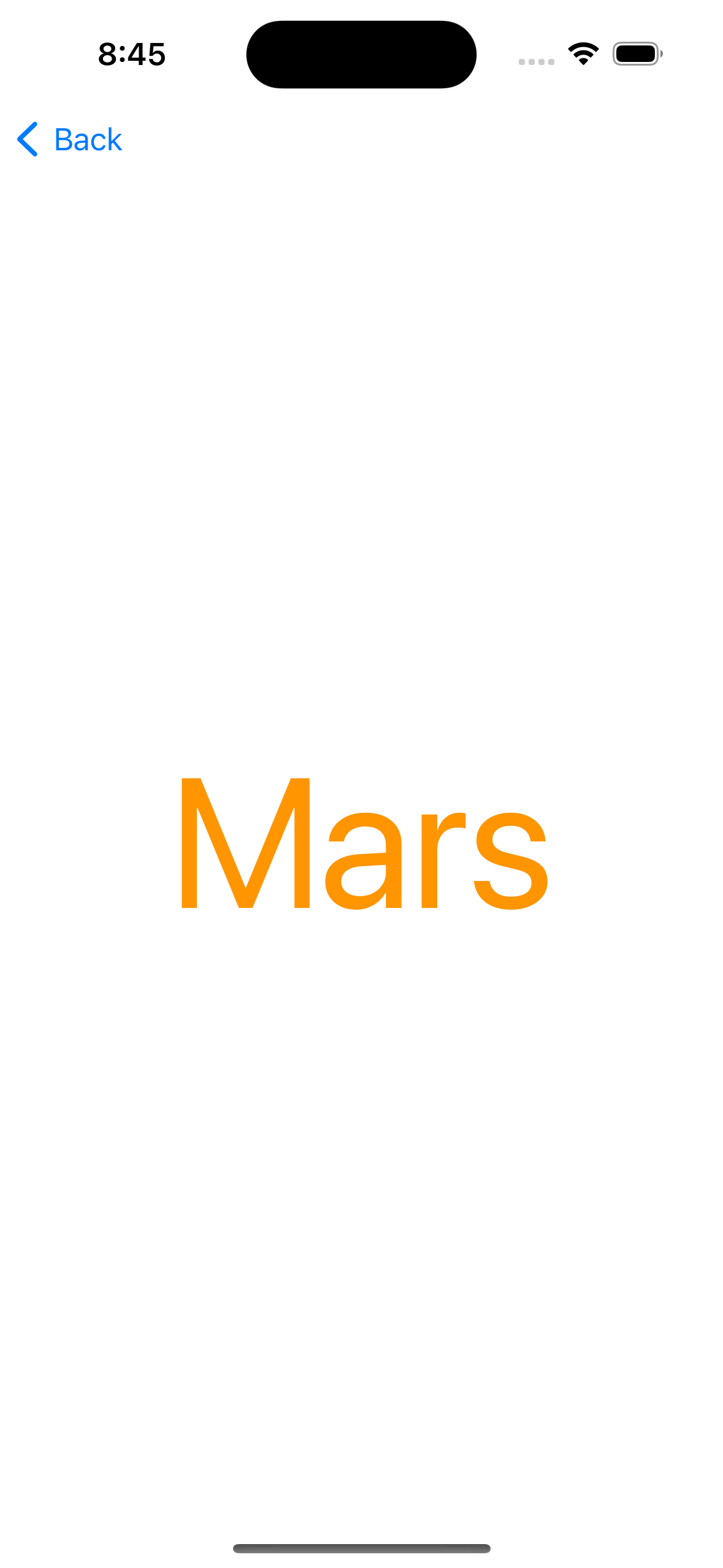 |
ContentView
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
import SwiftUI
struct ContentView: View {
//about GridItem https://developer.apple.com/documentation/swiftui/griditem/size-swift.enum
var columns: [GridItem] = [
GridItem(.flexible(minimum: 140)),
GridItem(.flexible()),
GridItem(.flexible()),
]
let cards: [Card] = MockStore.cards
var body: some View {
NavigationStack {
ScrollView(.vertical, showsIndicators: false) {
LazyVGrid(columns: columns, spacing: 16) {
ForEach(cards) { card in
NavigationLink(value: card) {
CardView(title: card.title)
.frame(height: 150)
}
.navigationDestination(for: Card.self) { card in
Text(card.title)
.foregroundColor(.orange)
.font(.system(size: 100))
}
}
}
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
|